XPath normalize-space example in Selenium WebDriver
In this example we will discuss about the usage of normalize-space
in Xpath.
We already aware of the Functions like text(),contains(),starts-with(),last() and position()
in Xpath. Refer to this link to know details about these functions. Xpath Functions.
Generally, normalize-space(String s)
is a method in Xpath useful to remove any leading or trailing spaces in a String. This is also works like a trim()
function in java.lang.String class. We will see different examples
<tr>
<td>
<a href="//money.rediff.com/companies/thomas-cook-i/16610005"> Thomas Cook (I) </a>
</td>
<td>A</td>
<td>244.60</td>
<td>252.95</td>
<td>
</tr>
In the above example the table data has spaces for Thomas Cook
. If we use Xpath Text Function it doesnt provide any value as an output. Please check the screenshot below:
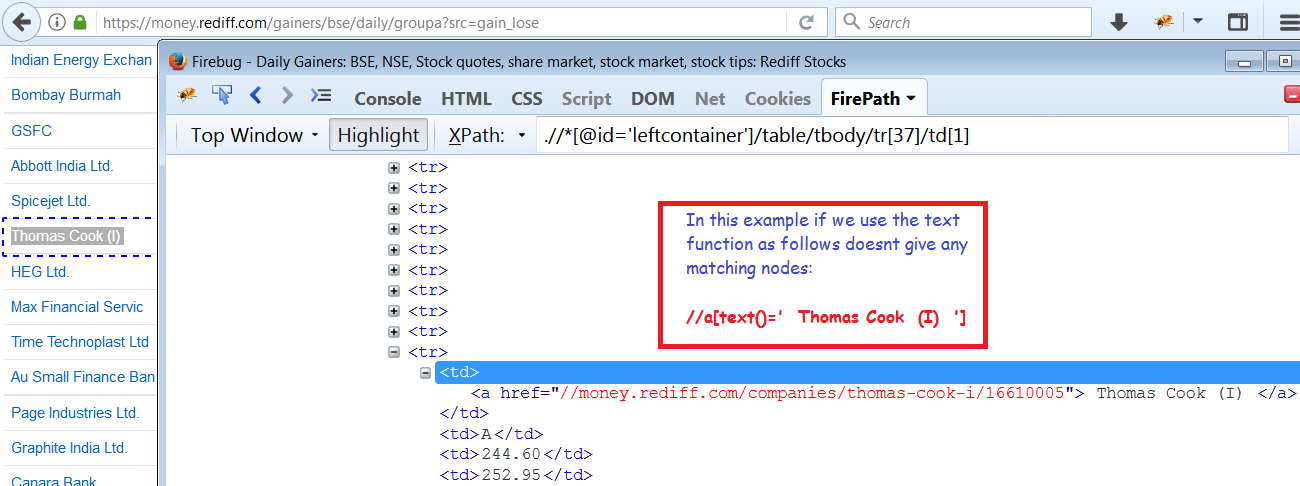
Usage of normalize-space(text()) method in Xpath.Please check the screenshot below:
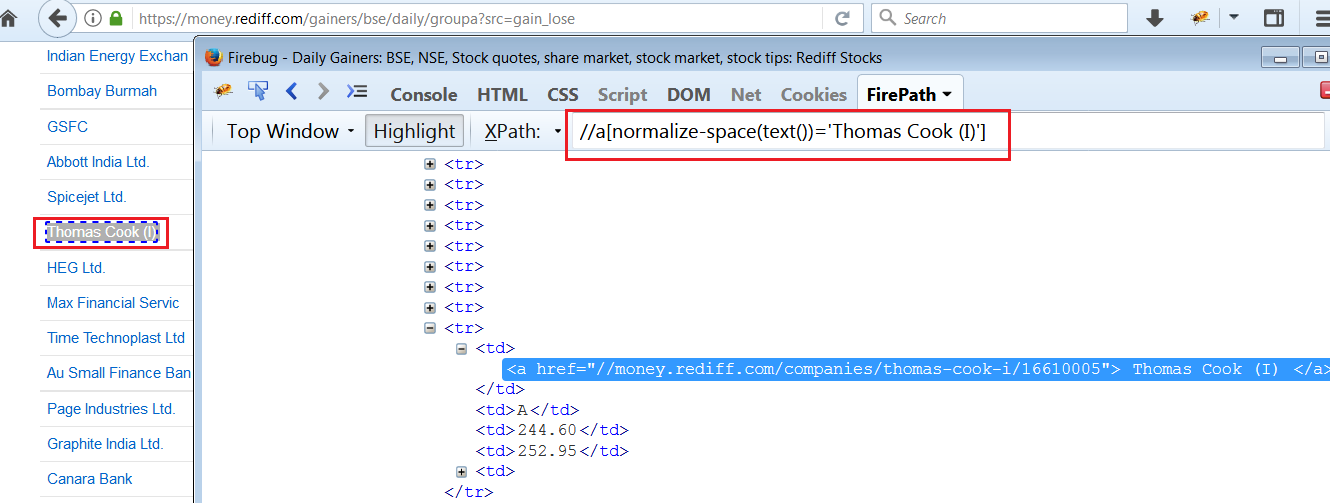
Selenium WebDriver Example to fetch the price for a particular Stock:
package org.totalqa.selenium;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
public class XpathAxesLocators {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "geckodriver.exe");
FirefoxDriver driver = new FirefoxDriver();
driver.get("https://money.rediff.com/gainers/bse/daily/groupa?src=gain_lose");
/**
* Solution 1 using Axes Locators
*/
WebElement e = driver.findElement(By.xpath("//a[normalize-space(text())='Thomas Cook (I)']/parent::td/following-sibling::td[2]"));
try{
if(e.isDisplayed())
{
String price = e.getText();
System.out.println("Stock Price" + price);
}
}
catch(NoSuchElementException ex)
{
System.out.println("Stock Name Not Exists"+ ex.getMessage());
}
/**
* Solution 2 using findElements()
*/
List<WebElement> trList = driver.findElements(By.xpath(".//*[@id='leftcontainer']/table/tbody/tr/td[1]/a"));
for(int i=0;i<trList.size();i++)
{
if(trList.get(i).getText().contains("Thomas Cook (I)"))
{
e = driver.findElement(By.xpath(".//*[@id='leftcontainer']/table/tbody/tr["+(i+1)+"/td[3]"));
System.out.println("Stock Price:::" + e.getText());
break;
}
}
}
}
Therefor the extra spaces in the table data is ignored and identified the element and fetches the stock price.
Output:
Stock Price::: 244.60
Conclusion:
In the example we have learnt how to use the normalize-space
in Xpath.